RFID Garage Door Opener
- Christian Stoldal
- Jan 22, 2021
- 2 min read
When my roommates and I moved into a new house we were renting we were only given one garage door opener between the four of us. The simple solution is to go buy 3 more garage door openers, I though it would be more interesting to create a RFID tag controlled garage door opener. This way all of us could simply have a RFID tag on our keychain or RFID sticker on our phones.
Materials Used
Arduino Nano
RFID RC522
2 x 9V battery
1 x RGB LED
Small Project box
Schematic
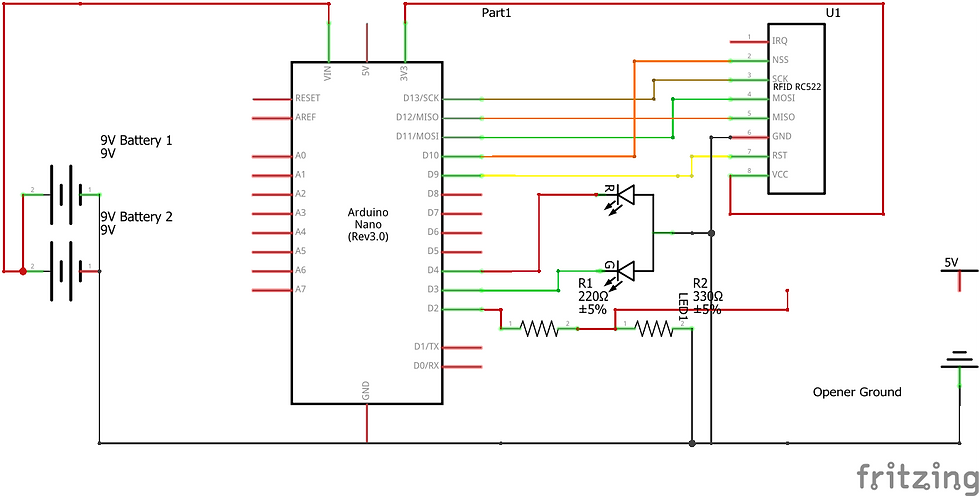
Code
#include <SPI.h>
#include <MFRC522.h>
#define SS_PIN 53
#define RST_PIN 5
MFRC522 mfrc522(SS_PIN, RST_PIN);
const int GreenLED = 9;
const int RedLED = 8;
const int ActivationPin = 2;
const int numberOfWhiteListedIDs = 5;
const String WhiteListedUID[numberOfWhiteListedIDs] = {"99 4C BD 5A","30 1A 0B 19","DE 00 0D 9B","CE E3 D9 AA","2E F7 0C 9B"};
String testUID = "99 4c BD 5A";
/** Function Defs */
void doorActivation();
bool UIDCheck(String tempUID);
String Byte2String();
/** Main */
void setup() {
Serial.begin(9600);
SPI.begin();
mfrc522.PCD_Init();
pinMode(ActivationPin, OUTPUT);
pinMode(GreenLED, OUTPUT);
pinMode(RedLED, OUTPUT);
digitalWrite(ActivationPin, HIGH);
}
void loop() {
String currentTAG;
bool access = 0;
if ( ! mfrc522.PICC_IsNewCardPresent())
{
return;
}
// Select one of the cards
if ( ! mfrc522.PICC_ReadCardSerial())
{
return;
}
currentTAG = Byte2String();
access = UIDCheck(currentTAG);
if (access == 1) {
doorActivation();
}
}
void doorActivation()
{
digitalWrite(GreenLED, HIGH);
Serial.print("Status LED: activated \n");
digitalWrite(ActivationPin, LOW);
Serial.print("Actvation Pin: activated \n");
delay(100);
Serial.print("Actvation Pin: deactivated \n");
digitalWrite(ActivationPin, HIGH);
delay(2000);
Serial.print("Status LED: deactivated \n");
digitalWrite(GreenLED, LOW);
};
bool UIDCheck(String tempUID){
Serial.print(" UID being test:");
Serial.print(tempUID);
Serial.println("-");
for (int i = 0; i < numberOfWhiteListedIDs; i++) {
Serial.print("Testing against: ");
Serial.print(WhiteListedUID[i]);
Serial.println("-");
if (tempUID.substring(1) == WhiteListedUID[i]) {
Serial.print(" ACESS GRANTED FOR UID: ");
Serial.println(tempUID);
return 1;
}
}
Serial.print("ACESS DENIED FOR UID: ");
Serial.println(tempUID);
digitalWrite(RedLED, HIGH);
delay(2000);
digitalWrite(RedLED, LOW);
return 0;
};
String Byte2String(){
String UniversalID = "";
byte letter;
for (byte i = 0; i < mfrc522.uid.size; i++)
{
UniversalID.concat(String(mfrc522.uid.uidByte[i] < 0x10 ? " 0" : " "));
UniversalID.concat(String(mfrc522.uid.uidByte[i], HEX));
}
UniversalID.toUpperCase();
return UniversalID;
}
Comments